Decentralized applications, or dApps, are becoming increasingly popular as they offer a more secure and transparent way of doing things on the internet. NEAR Protocol is one such blockchain platform that allows developers to create and deploy apps that can run on a decentralized network. In this tutorial, we will guide you through the steps to the NEAR dApp tutorial.
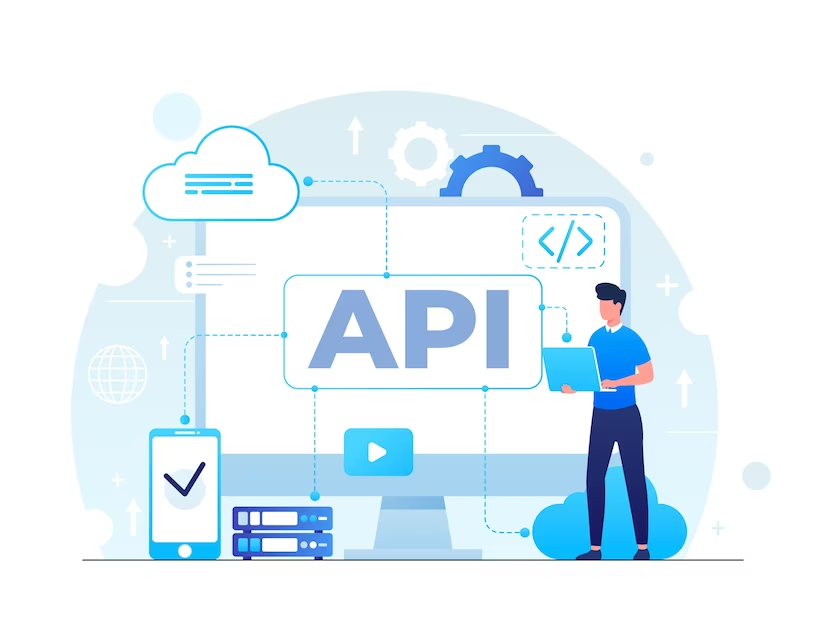
Step 1: Set up your NEAR Wallet and TestNet
Before we start, you will need to set up a NEAR Wallet and connect to the TestNet network. This will allow you to test your dApp on a sandbox environment before deploying it on the main network.
To set up your NEAR Wallet, head to wallet.near.org and follow the instructions to create a new wallet. Once you have created your wallet, you can connect to the TestNet network by changing the network settings in your wallet. This will allow you to receive and send test tokens on the TestNet.
Step 2: Install the NEAR CLI
The NEAR CLI is a command-line interface tool that allows you to interact with the NEAR Protocol. You will need to install the NEAR CLI to create and deploy your dApp on the platform.
To install the NEAR CLI, open your terminal and enter the following command:
```
npm install -g near-cli
```
This will install the NEAR CLI globally on your system.
Step 3: Create your dApp project
Once you have set up your wallet and installed the NEAR CLI, you can start creating your dApp project. For this tutorial, we will create a simple dApp that allows users to store and retrieve messages on the blockchain.
To create your project, open your terminal and enter the following command:
```
near create-app my-dapp
```
This will create a new folder named `my-dapp` in your current directory, containing the basic structure of your dApp project.
Step 4: Write and deploy your smart contract
Now that you have created your dApp project, you can start writing your smart contract. Smart contracts are the backbone of dApps and are responsible for executing the logic of your application on the blockchain.
To write your smart contract, navigate to the `my-dapp` folder in your terminal and open the `src` folder. Here, you will find a file named `lib.rs`. This is where you will write the code for your smart contract.
For our message storage dApp, we will write a simple smart contract that allows users to set and get a message on the blockchain. Here's the code:
```
use borsh::{BorshDeserialize, BorshSerialize};
use near_sdk::{env, near_bindgen};
#[near_bindgen]
#[derive(Default, BorshDeserialize, BorshSerialize)]
pub struct MyContract {
message: String,
}
#[near_bindgen]
impl MyContract {
pub fn set_message(&mut self, message: String) {
self.message = message;
}
pub fn get_message(&self) -> String {
self.message.clone()
}
}
```
This smart contract defines a `MyContract` struct that stores a `message` field. It also has two methods `set_message` and `get_message` that allow users to set and retrieve the message on the blockchain.
Once you have written your smart contract code, you can deploy it to the TestNet network using the following command:
```
near deploy --wasmFile res/my_contract.wasm --accountId my-dapp.testnet
```
This will deploy your smart contract to the TestNet network and create a new account for your dApp with the account ID `my-dapp.testnet`.
Step 5: Create your dApp frontend
Now that you have deployed your smart contract, you can create the frontend of your dApp. This is the user interface that users will interact with to use your application.
For this tutorial, we will create a simple frontend that allows users to set and get the message on the blockchain.
To create your frontend, navigate to the `my-dapp` folder in your terminal and open the `src` folder. Here, you will find a file named `index.html`. This is where you will write the code for your frontend.
Here's the code for our message storage dApp frontend:
```
<!DOCTYPE html>
<html>
<head>
<meta charset="UTF-8">
<title>My dApp</title>
<script src="./index.js"></script>
</head>
<body>
<h1>My dApp</h1>
<label for="message">Message:</label>
<input type="text" id="message">
<button onclick="setMessage()">Set message</button>
<button onclick="getMessage()">Get message</button>
<p id="result"></p>
</body>
</html>
```
This HTML code creates a simple form with a text input and two buttons for setting and getting the message on the blockchain. It also has a paragraph element for displaying the result of the user's actions.
To make the frontend functional, we need to create a JavaScript file named `index.js` in the `src` folder and write the code for our dApp's logic. Here's the code:
```
import * as nearAPI from 'near-api-js';
const CONTRACT_NAME = 'my-dapp.testnet';
const provider = new nearAPI.providers.JsonRpcProvider('https://rpc.testnet.near.org');
const nearConfig = {
networkId: 'testnet',
nodeUrl: 'https://rpc.testnet.near.org',
contractName: CONTRACT_NAME,
walletUrl: 'https://wallet.testnet.near.org',
};
const keyStore = new nearAPI.keyStores.BrowserLocalStorageKeyStore();
const near = new nearAPI.Near({
networkId: nearConfig.networkId,
nodeUrl: nearConfig.nodeUrl,
deps: { keyStore: keyStore },
});
const contract = new nearAPI.Contract(
new nearAPI.Account(near.connection, CONTRACT_NAME),
CONTRACT_NAME,
{
viewMethods: ['get_message'],
changeMethods: ['set_message'],
sender: new nearAPI.Account(near.connection, CONTRACT_NAME),
}
);
window.setMessage = async () => {
const message = document.getElementById('message').value;
await contract.set_message({ message });
document.getElementById('result').innerText = `Message set to: ${message}`;
};
window.getMessage = async () => {
const message = await contract.get_message();
document.getElementById('result').innerText = `Current message: ${message}`;
};
```
This JavaScript code connects to the TestNet network using the NEAR API and defines the logic for setting and getting the message on the blockchain. It also exports two functions `setMessage` and `getMessage` that can be called from the HTML file to interact with the smart contract.
Step 6: Deploy your dApp
Once you have created your smart contract and frontend, you can deploy your dApp to the TestNet network using the following command:
```
near deploy --accountId my-dapp.testnet --wasmFile res/my_contract.wasm --initFunction new --initArgs '{"owner_id": "my-dapp.testnet"}'
```
This will deploy your dApp to the TestNet network and provide you with the contract ID.
Copy the contract ID and navigate to the `src` folder in your terminal. Here, you can open the `index.html` file and replace the `CONTRACT_NAME` variable with your contract ID.
Save the changes to the file and run a local server to preview your dApp by running the following command:
```
npm run start
```
This will start a local server at `http://localhost:1234`. Navigate to this URL in your browser to see your dApp in action!
Step 7: Test your dApp
To test your dApp, you can set and get the message using the frontend form. When you set the message, it will be stored on the blockchain and can be retrieved later by clicking the "Get message" button.
Congratulations, you have successfully created a NEAR dApp that stores data on the blockchain!
Conclusion
NEAR is a powerful blockchain platform that makes it easy for developers to create decentralized applications. By following the steps outlined in this tutorial, you can create your own NEAR dApp and deploy it to the TestNet network.
Remember to follow best practices when developing your dApp, such as writing secure smart contracts and testing your code thoroughly before deployment.
With the growing popularity of decentralized applications, now is the perfect time to get started with NEAR and start building your own dApps. Happy coding!
Comments